Digital Filtering: Software
In order to provide an implementation example of digital filters, GNC Tools provides the following filter software functionality:
- Discrete
- Required to be practically implementable on an embedded system.
- FIR/IIR
- Functionality for both FIR and IIR filters up to 6th order.
- SOS
- Functionality for Direct Form II Second Order Section filters up to 6 sections.
- Native C
- No external libraries other than the C standard library.
- C++ Wrapper
- C++ wrappers included with all functionality.
- Documented
- Fully documented source code suitable for Doxygen auto-generated output.
- Unit Tested
- Validated against the MATLAB Signal Processing Toolbox digitalFilter class.
Clone GNCTools to access the filter source, including MEX interfaces and MATLAB examples and unit tests, or alternatively jump into the Filter Source Code to have a look! The examples via the links above can be found in Control/Filter/Filter_Examples.m
.
FIR Filtering
An FIR filter can be simulated by using the MEX interface to the C implementation mxCFilter
or the C++ wrapper mxFilter
.
N = 6;
b = (1/(N+1))*ones(1,(N+1));
a = 1;
% Initialize MEX interface to C Filter
mxCFilter('Init',b,a)
% Filter input sine wave
Fs = 100;
t = 0:(1/Fs):0.5;
u = sin(5*2*pi*t); % 5Hz Input
y = mxCFilter('Update',u);
% Plot Output
plot(t,u,t,y)
grid on; xlabel('Time [s]'); ylabel('Magnitude')
legend('Input','Output')
title('FIR Example: Moving Average Filter')
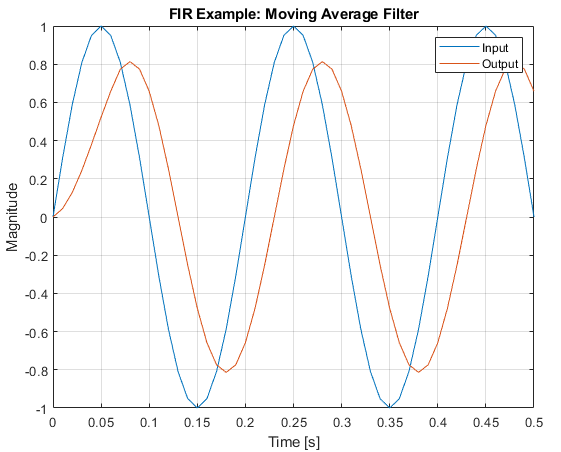
IIR Filtering
An IIR filter can be simulated by using the MEX interface to the C implementation mxCFilter
or the C++ wrapper mxFilter
.
Fs = 100;
Fc = 5; % Corner Freq [Hz]
N = 2;
h = fdesign.highpass('N,F3dB', N, Fc, Fs);
Hd = design(h, 'butter');
[b,a] = tf(Hd);
% Initialize MEX interface to C Filter
mxCFilter('Init',b,a)
% Filter input sine wave
t = 0:(1/Fs):4;
u = sin(2*2*pi*t); % 2Hz Input
y = mxCFilter('Update',u);
% Plot Output
plot(t,u,t,y)
grid on; xlabel('Time [s]'); ylabel('Magnitude')
legend('Input','Output')
title('IIR Example: High Pass Filter')
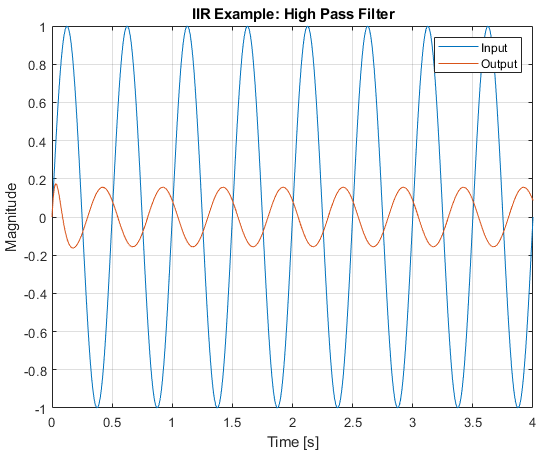
SOS Filtering
A Cascaded Section Order Section filter can be simulated by using the MEX interface to the C implementation mxCSOSFilter
or the C++ wrapper mxSOSFilter
.
Fs = 100;
Fc1 = 0.8; % First Cutoff Frequency
Fc2 = 1.25; % Second Cutoff Frequency
N = 2;
h = fdesign.bandstop('N,F3dB1,F3dB2', N, Fc1, Fc2, Fs);
Hd = design(h, 'butter');
% Initialize MEX interface to C SOS Filter using Filter Object Directly
mxCSOSFilter('Init',Hd)
% or via the coefficient structure
mxCSOSFilter('Init',Hd.coeffs)
% or via the SOS Matrix and Gain Vector individually
mxCSOSFilter('Init',Hd.sosMatrix, Hd.ScaleValues)
% Filter input sine wave
t = 0:(1/Fs):4;
u = sin(1*2*pi*t); % 1Hz Input
y = mxCFilter('Update',u);
% Plot Output
plot(t,u,t,y)
grid on; xlabel('Time [s]'); ylabel('Magnitude')
legend('Input','Output')
title('SOS Example: Notch Filter')
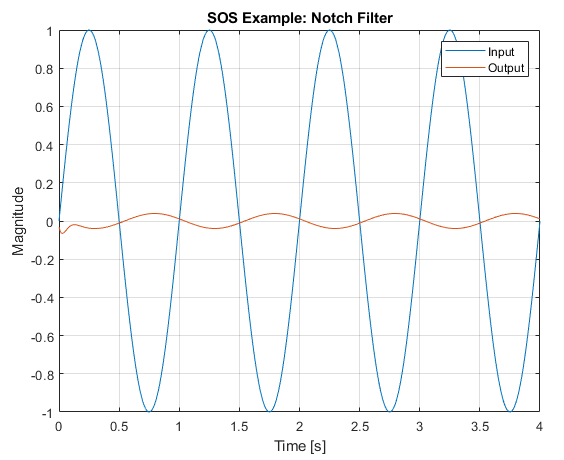